- Published at
Jest conditionally mocking functions
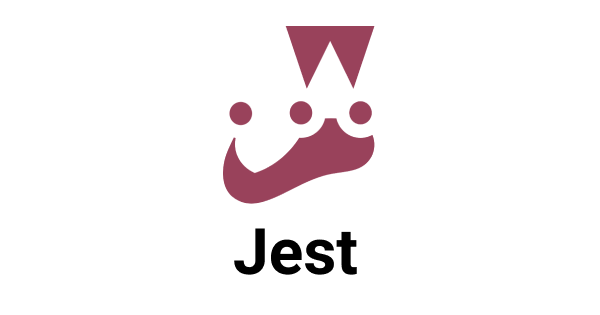
Reference implementation for mocking any function using Jest
- Authors
-
-
- Name
- Joachim Bülow
- Cofounder and CTO at Doubble
-
Table of Contents
Conditionally mocking functions with Jest
Mocking with Jest can be really easy, and sometimes really tricky.
Recently, I wanted to mock only one specific database call in a test suite, and otherwise have my database work as usual in one of our end to end tests.
Here’s how I did it.
Defining the original function
In my setup, i want to conditionally mock the query
function on my context.connection
(TypeOrm connection) object.
I extract this into a constant like so:
const originalQueryImplementation = context.connection.query;
Mocking the function conditionally
With the original function saved, we can use the jest.spyOn
function to mock the implementation.
In my case, i want to mock that a user has “swiped” an average of 1000 swipes daily recently.
const originalQueryImplementation = context.connection.query;
jest.spyOn(context.connection, 'query').mockImplementation((sql, ...args) => {
if (sql.includes('average_swipes')) {
return Promise.resolve([{ average_swipes: 1000 }]);
}
return originalQueryImplementation.apply(context.connection, [sql, ...args]);
});
Notice how i can conditionally check the contents of the arguments, to not interfere with other calls to
query
. If the aguments to not match, i can apply
the captured arguments onto the original function implementation and return.
I also pass in the original connection object as the this
context to the original function.
Yay!